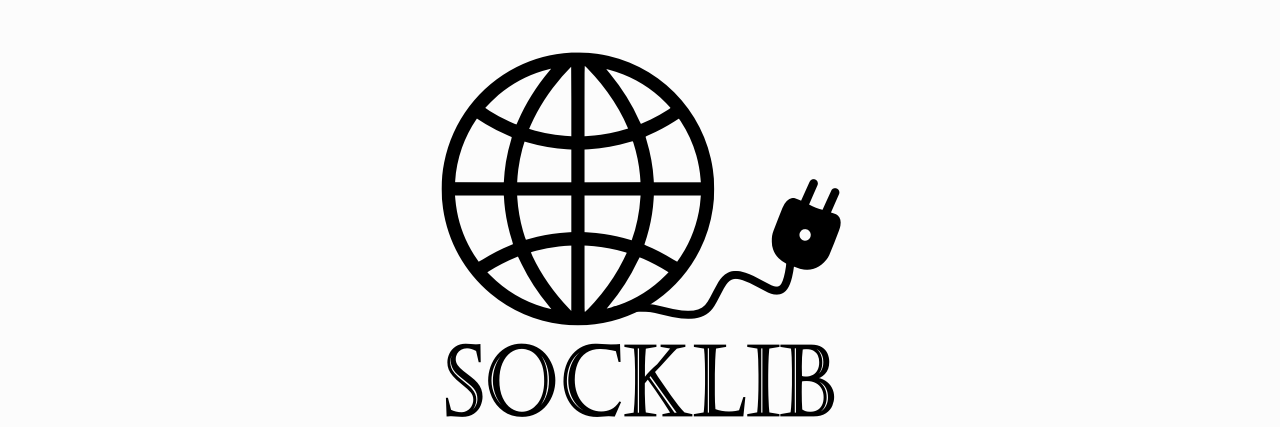
socklib
is an open source C++ library that aims to provide a Platform agnostic Python-Like Socket
object. The object give
you access to the BSD socket interface by creating a wrapper around C interface, while also adding
some more methods inspired by Python's socket
module.
Requirements
To be able to use socklib
, you will need a modern compiler that supports C++17.
The following requirements are also necessary:
premake5
with the ability to run it from the command line.make
(on Linux)
premake5
here.
Build & Testing
Start by clonning the repository with: git clone https://github.com/VMormoris/socklib
.
Then depending on the platform you use, you have to generate and build the project following those steps:
-
On Windows:
premake5 vs20XX
(where XX the version of Visual Studio you have).- Open the solution file that was generated or use
msbuild
in order to build the library and run the tests
-
On Linux:
premake5 gmake2
make
to build the library and the tests./bin/Debug-linux/tests/tests
to run the tests
Using
In order to use the library on your own project you will need:
- Add the
include
folder as an include directory on your project - Link your project with the apropriate
socklib.lib
file (Debug or Release)
Examples
An example of an echo server usingsocklib
:
#include <socklib/Socket.h>
// ------- Only at the Entry Point file -------
#define SOCK_MAIN
#include <socklib/Platform.h>
// --------------------------------------------
using namespace socklib;
int main(int argc, char** argv)
{
Socket server(AF_INET, SOCK_STREAM, 0);
server.Bind("127.0.0.1", 55555);
server.Listen();
char buffer[KiB];
while(true)
{
auto [sock, client] = server.Accept();
const auto& [address, port] = client;
int bytes = sock.Receive(buffer, KiB);
bytes = sock.Send(buffer, bytes);
sock.Close();
}
server.Close();
return 0
}
An example of a Client that connect a send a message to the server above:
#include <socklib/Socket.h>
// ------- Only at the Entry Point file -------
#define SOCK_MAIN
#include <socklib/Platform.h>
// --------------------------------------------
using namespace socklib;
int main(int argc, char** argv)
{
constexpr char msg[] = "Hello from socklib";
char buffer[KiB];
Socket sock(AF_INET, SOCK_STREAM, 0);
sock.Connect("127.0.0.1", 55555);
int bytes = sock.Send(msg, 19);
bytes = sock.Receive(buffer, KiB);
sock.Disconnect();
sock.Close();
return 0;
}
Third Party Libraries
Contributing
- Fell free to contribute to any of the open issues or open a new one describing what you want to do.
- For small scale improvements and fixes simply submit a GitHub pull request.